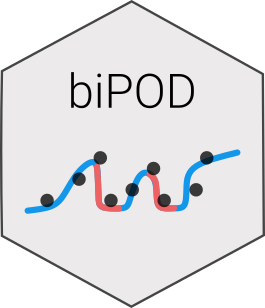
Simulate Population Growth with Stochastic Logistic Dynamics
sim_stochastic_logistic.Rd
Simulates population growth over multiple time steps using a stochastic logistic growth model. This model incorporates both stochastic elements and a carrying capacity to describe population dynamics. Population size changes due to random births and deaths, but the growth rate is constrained by the carrying capacity \(K\).
Arguments
- n0
Numeric value indicating the initial population size at time 0. This must be a non-negative integer (>= 0).
- lambda
Numeric vector or scalar specifying the birth rates at each time step. If a scalar is provided, it is assumed to be constant for all time steps. If a vector is provided, it should be of length
steps
, specifying the birth rate at each step.- mu
Numeric vector or scalar specifying the death rates at each time step. Like
lambda
, if a scalar is provided, it is assumed to be constant for all time steps. If a vector is provided, it should be of lengthsteps
, specifying the death rate at each step.- K
Numeric value indicating the carrying capacity of the environment. This must be a non-negative integer (> 0).
- steps
Integer specifying the number of time steps to simulate. Must be a non-negative integer.
- delta_t
Numeric vector or scalar specifying the time step sizes. If a scalar is provided, it is assumed to be constant for all steps. If a vector is provided, it should be of length
steps
, specifying the time step size at each step.
Value
A tibble
with the following columns:
time
: The cumulative time at each step, starting from 0.count
: The population size at each time step, updated based on the stochastic logistic model.group
: A factor that groups contiguous intervals where the birth and death rates are constant (i.e.,lambda
andmu
remain the same over consecutive time steps).
Details
This function simulates a population following a stochastic logistic growth model, where the population size changes due to random births and deaths while being constrained by the carrying capacity \(K\).
The population's growth rate decreases as the population size approaches \(K\), representing the limitations of resources. The rates of birth (lambda
) and death (mu
) may vary over time.
The stochastic component of the model introduces randomness, making the population size fluctuate between time steps.
At each time step, the birth and death rates are used to calculate the expected population change, and a random event (birth or death) is chosen based on the probabilities at that step. The population is updated accordingly.
The simulation stops when the population reaches zero or when the specified number of steps is completed.
If steps
is set to 0, the function returns the initial population size n0
immediately, without any simulation.
Examples
# Simulate a stochastic logistic growth process with constant rates and carrying capacity of 100
sim_stochastic_logistic(n0 = 10, lambda = 0.2, mu = 0.1, K = 100, steps = 15, delta_t = .25)
#> # A tibble: 16 × 3
#> time count group
#> <dbl> <dbl> <dbl>
#> 1 0 10 0
#> 2 0.25 11 0
#> 3 0.5 12 0
#> 4 0.75 11 0
#> 5 1 8 0
#> 6 1.25 7 0
#> 7 1.5 9 0
#> 8 1.75 8 0
#> 9 2 9 0
#> 10 2.25 10 0
#> 11 2.5 12 0
#> 12 2.75 13 0
#> 13 3 12 0
#> 14 3.25 15 0
#> 15 3.5 13 0
#> 16 3.75 15 0
# Simulate with varying rates
lambda_rates <- c(rep(0.3, 5), rep(0.1, 5), rep(0.9, 5))
mu_rates <- c(rep(0.1, 5), rep(0.15, 5), rep(0.3, 5))
sim_stochastic_logistic(
n0 = 10,
lambda = lambda_rates,
mu = mu_rates,
steps = 15,
delta_t = .1,
K=200
)
#> # A tibble: 16 × 3
#> time count group
#> <dbl> <dbl> <dbl>
#> 1 0 10 0
#> 2 0.1 12 0
#> 3 0.2 13 0
#> 4 0.3 15 0
#> 5 0.4 16 0
#> 6 0.5 16 0
#> 7 0.6 15 1
#> 8 0.7 15 1
#> 9 0.8 14 1
#> 10 0.9 12 1
#> 11 1 11 1
#> 12 1.1 12 2
#> 13 1.2 12 2
#> 14 1.3 14 2
#> 15 1.4 18 2
#> 16 1.5 21 2